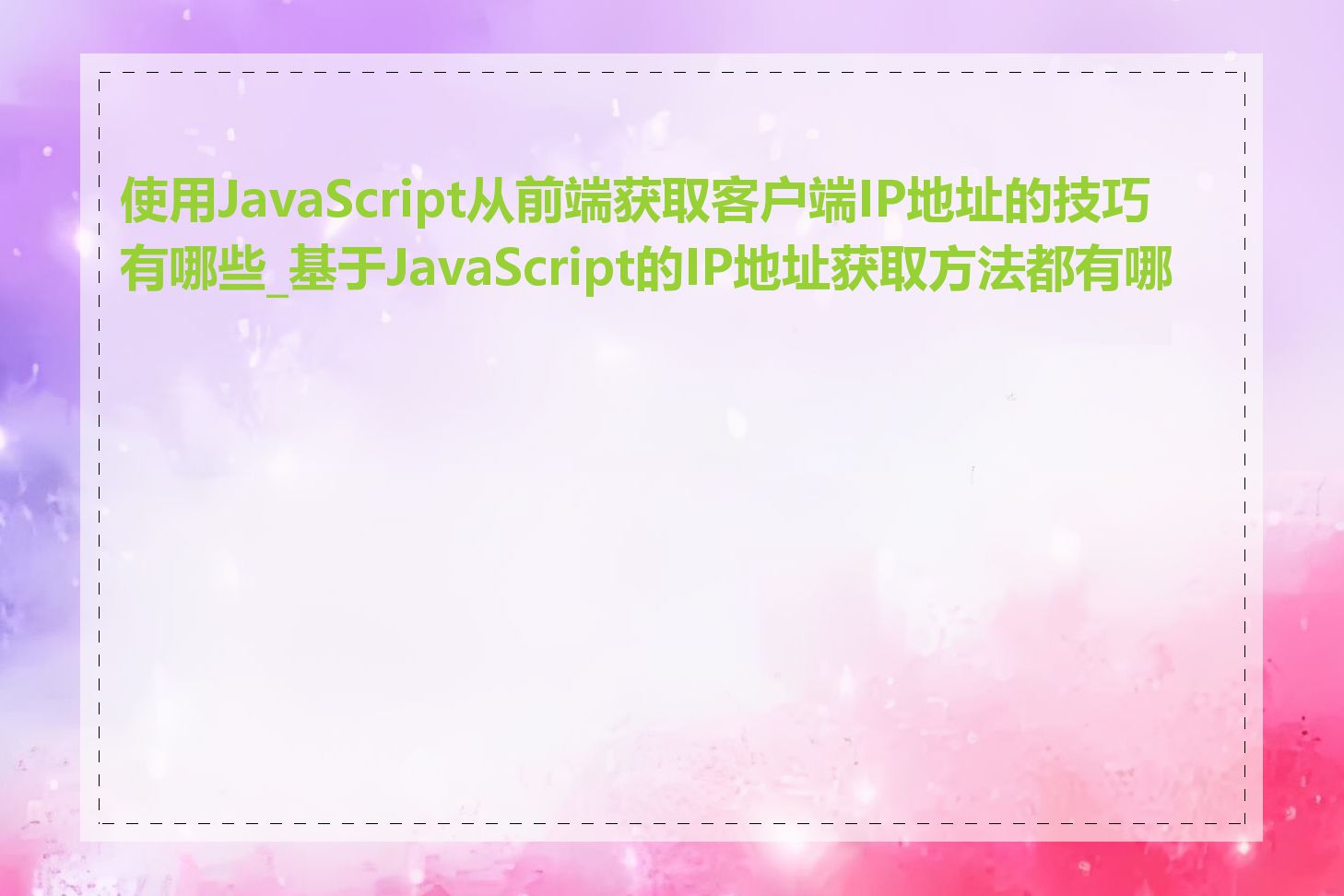
在前端开发中,有时需要获取客户端的IP地址信息。这可以用于多种场景,例如统计分析、安全性检查、地理位置定位等。那么,使用JavaScript从前端获取客户端IP地址有哪些技巧呢?
1.
WebRTC API
WebRTC(Web Real-Time Communication)是一种基于浏览器的实时通信技术。通过WebRTC的 RTCPeerConnection API,可以获取客户端的本地IP地址。示例代码如下:
function getIP(onNewIP) { // onNewIp - your listener function for new IPs
//compatibility for firefox and chrome
var myPeerConnection = window.RTCPeerConnection || window.mozRTCPeerConnection || window.webkitRTCPeerConnection;
var pc = new myPeerConnection({
iceServers: []
}),
noop = function() {},
localIPs = {},
ipRegex = /([0-9]{1,3}(\.[0-9]{1,3}){3}|[a-f0-9]{1,4}(:[a-f0-9]{1,4}){7})/g;
function iterateIP(ip) {
if (!localIPs[ip]) onNewIP(ip);
localIPs[ip] = true;
}
//create a bogus data channel
pc.createDataChannel("");
// create offer and set local description
pc.createOffer(pc.setLocalDescription.bind(pc), noop);
// gather candidates
pc.addEventListener('icecandidate', function(ice) {
if (!ice || !ice.candidate || !ice.candidate.candidate) return;
var myIP = /([0-9]{1,3}(\.[0-9]{1,3}){3}|[a-f0-9]{1,4}(:[a-f0-9]{1,4}){7})/.exec(ice.candidate.candidate)[1];
iterateIP(myIP);
});
}
// Usage
getIP(function(ip){ console.log('got ip: ', ip); });
2.
JSONP方式
通过JSONP方式,可以从第三方服务获取客户端IP地址信息。示例代码如下:
function getIP(callback){
var script = document.createElement('script');
script.type = 'text/javascript';
script.src = 'https://api.ipify.org?format=jsonp&callback=getIP';
var head = document.getElementsByTagName('head')[0];
head.appendChild(script);
}
// Usage
getIP(function(ip){
console.log('My public IP address is: ', ip);
});
3.
服务端渲染
网站使用服务端渲染,则可以在服务端获取客户端的IP地址,将其传递给前端。示例代码如下(Node.js):
const http = require('http');
const server = http.createServer((req, res) => {
const clientIP = req.headers['x-forwarded-for'] || req.connection.remoteAddress;
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ ip: clientIP }));
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
以上就是一些基于JavaScript的IP地址获取方法,每种方式都有各自的优缺点。开发者可以根据具体需求选择合适的方式。
本文介绍从前端获取客户端IP地址的几种主要技巧,包括利用WebRTC API、JSONP方式以及服务端渲染等。这些方法各有优劣,开发者可以根据实际需求选择合适的方式。通过JavaScript从前端获取客户端IP地址是一个很有用的技能,可以应用于各种场景中。